A few months ago, our colleagues complained that our project was slow to build: it normally took 5 minutes, especially in branch switching – which took about 10 minutes. After that, we realized – it doesn’t happen uniquely to our project, it’s a common issue. So here are a few things we did in our Android project to improve the situation.
Profiling
How long does it take to get your build done? Add the — profile param to get the exact number. In Android Studio, go to Preferences -> Build, Execution, Deployment -> Compiler and set:
Command-line Options: --profile
Let build. You could file a HTML file in [project_dir]/build/reports/profile/ generated with the detailed time spent for each build task as follows:
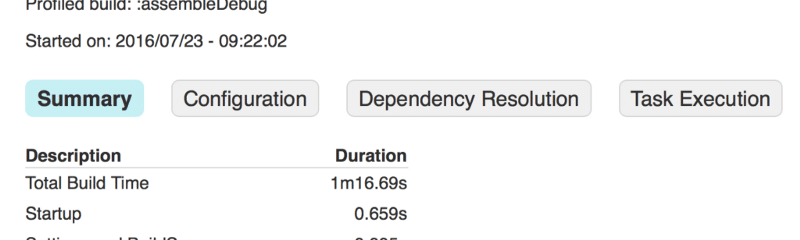
We recommend you see the report for any optimization we do in this article to see how good each parameter is.
Reasons
There are some common causes of the slow build:
Repositories and dependencies
What does Android Studio do when you click on “Sync Gradle” (if build.gradle file is changed)or create a project? Answer: Gradle collects all the dependencies. The good news is that Gradle uses cache, a folder that stores all dependencies to prevent them from going online. The bad news is, if Gradle cannot find any specific dependency, it will always go online to scan the repositories (usually mavenCenter or jcenter); so it will be prolonged if the network isn’t good enough.
There are 2 reasons why Gradle cache cannot be used:
- Dependency version isn’t fixed.
You can use + signal to let Gradle check for the latest version of a dependency since it always gets Gradle online.
compile 'com.google.code.gson:gson:2.+'
- Gradle version isn’t fixed.
Each Gradle organizes the cache itself and does not share the dependency cache. If your team has a few Gradle versions, it will take time to switch and download all the dependencies when you switch between Gradle versions.
Gradle isn’t optimised
Gradle provides several parameters that allow us to optimize but often they are not set in Android Studio.
Gradle is slow itself
Gradle is officially used and supported by the Android Team but it’s not the fastest build tool.
Solutions
The solutions to these issues are:
Setup the fixed Gradle and dependency versions
- Use same Gradle version as your team settings. A good way is to use Gradle wrapper instead of local Gradle by creating the gradlew, checking the source code repository, and going to Preferences -> Build, Execution, Deployment -> Build Tools -> Gradle
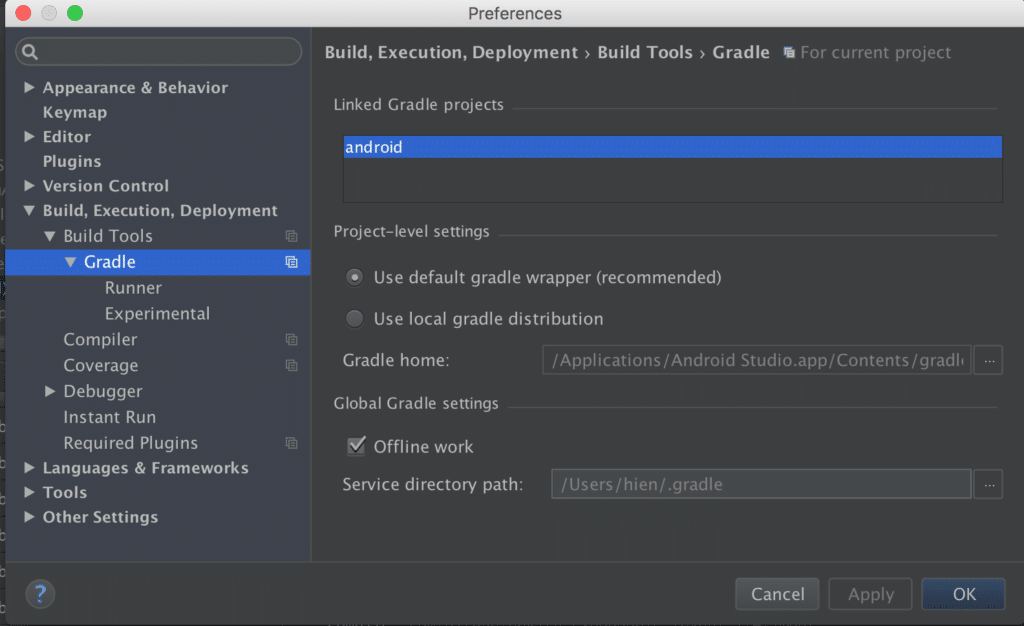
- Specific all the dependency version
compile 'com.google.code.gson:gson:2.4'
Use correct Gradle params
There are some Gradle params you could miss:
- — offline: Force Gradle using cache
- — configure-on-demand: Instead of all projects, let Gradle only build the relevant ones
- — daemon: Let Gradle use in-process build and cut the initial and warm-up state
- — parallel: Let Gradle build your projects in parallel, if possible
- — max-workers: Going with — parallel param to let Gradle know how many threads it can use
So typically, the command line call would be:
$ ./gradlew :assembleDebug --offline --configure-on-demand --daemon --parallel --max-workers=8 --profile
Mapping to Android Studio config, they would be:
Go to Preferences -> Build, Execution, Deployment -> Compiler
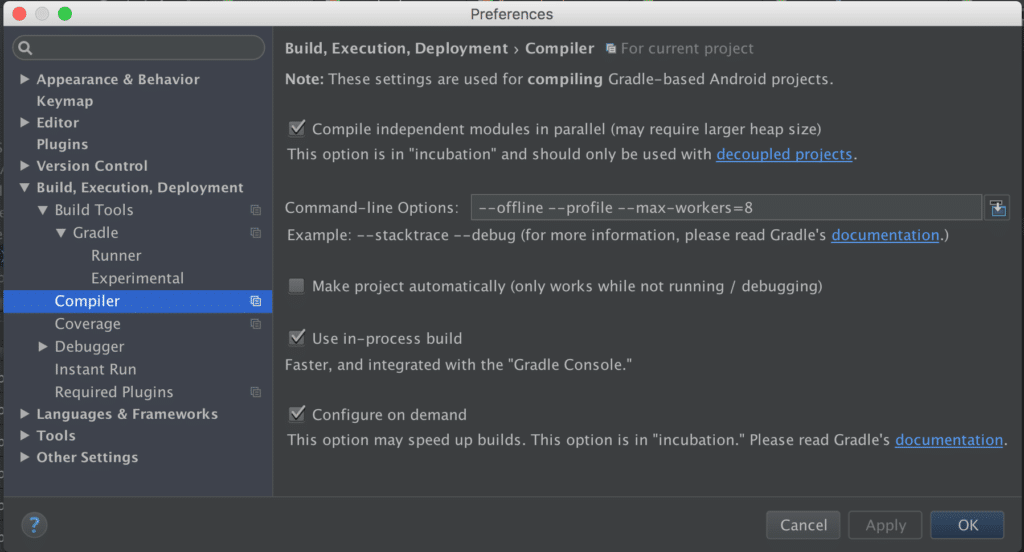
Go to Preferences -> Build, Execution, Deployment -> Build Tools -> Gradle
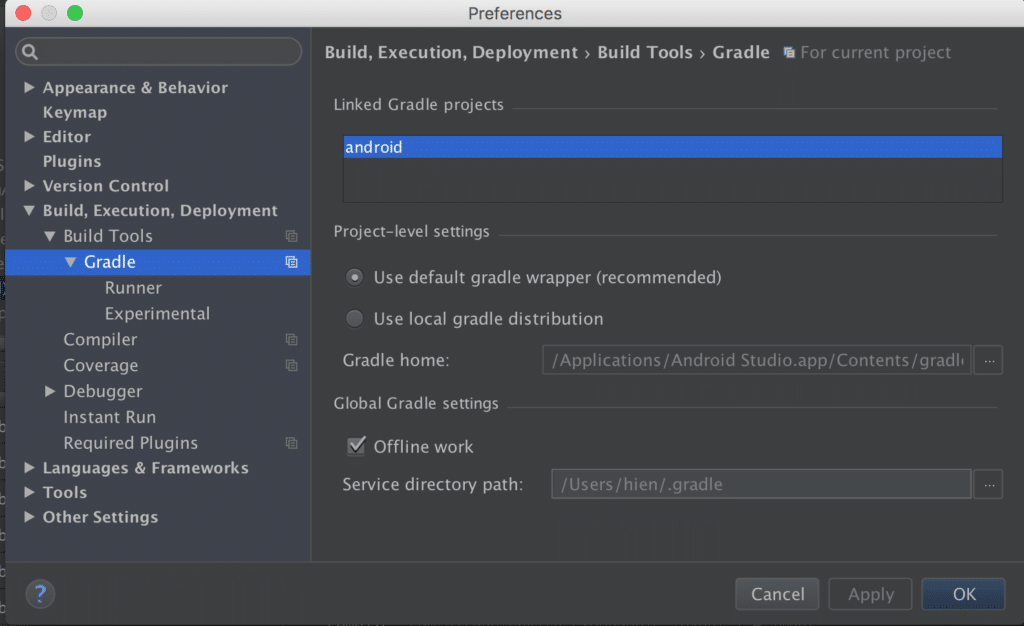
Other settings
Other settings could help is increasing the heap size for
- JVM
org.gradle.jvmargs = -Xmx5048m
- multiDex
dexOptions {
javaMaxHeapSize "4g"
jumboMode = true
preDexLibraries = false
incremental true
}
Use another build tool
It’s high time we built the project – Start by opening the HTML report file to see how much time we saved.
During the study, we found out that there are multiple tools that build faster than Gradle, namely Buck which is used by Facebook. On our demo, it’s incredibly fast. But of course, it isn’t supported by Android Team and its community is also small. The idea of keeping Gradle and Buck building in parallel is nice – stability provided by Gradle, speed by Buck.
We will return with another post regarding this topic soon at this location.