In this article, we will examine the best approaches for ReactJS state management and demonstrate how to maintain control over the state of your application. We shall see how ReactJS allows us to make a highly functionable app that benefits us to achieve our goals.
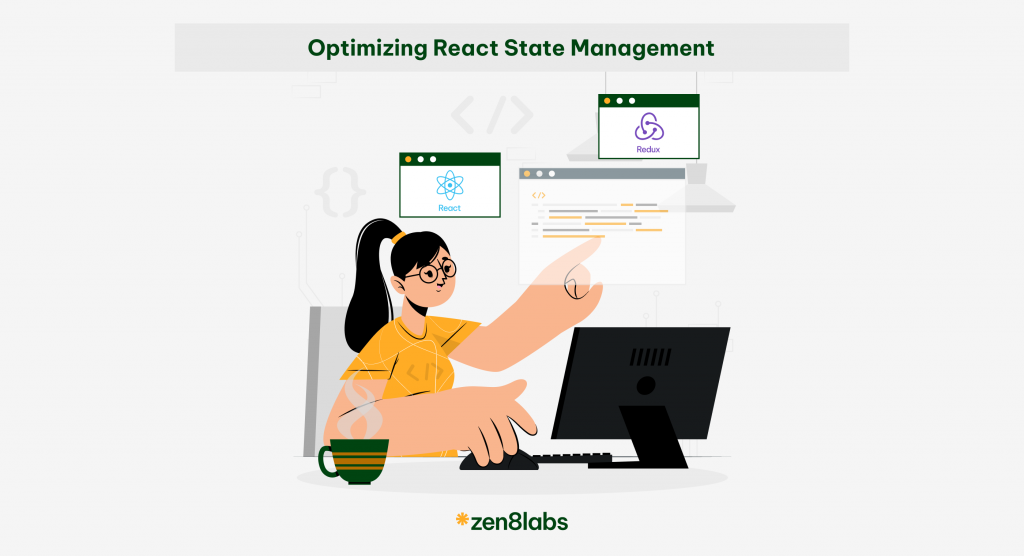
What makes state management critical?
State represents the data that changes over time in your application, and properly managing it ensures your app functions correctly. It helps you avoid common pitfalls like data inconsistency, prop drilling, and unnecessary re-renders. Understanding and effectively managing state is pivotal to ensuring your app is more maintainable, scalable, and performant.
Recognizing local component states
In ReactJS, state is managed within components using the useState or useReducer hooks. It is essential to understand the local component state and how it differs from global state management libraries like Redux, Recoil.
A local state should be used for data that is specific to a single component and does not need to be shared across the entire application. For complex apps with multiple components, consider using a global state management solution.
import * as React from "react";
const Component = () => {
const [localState, setLocalState] = React.useState();
return (
<>
Render local State
</>
);
}
Keep state close to where it’s used
A good practice is to keep the state as close to the components that need it as possible. This approach is known as “lifting state up,” reduces complexities and makes your app easier to reason about.
If multiple components need access to the same state, lift that state up to their nearest common ancestor. By doing so, you create a singular source of truth for that state, making it easier to manage and update.
import React, { useState } from 'react';
const Counter = () => {
// Local state for the count within the Counter component
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count - 1);
};
return (
<div>
<h2>Counter: {count}</h2>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default Counter;
Use immutability for state updates
It is fundamentally, good practice in React to never directly modify state. Instead, employ immutable data structures and techniques for state updates. Popular tools for achieving immutability include Immutable.js, Immer, and the spread operator.
These options allow you to create new state objects without altering the original. Adhering to this practice ensures that your state updates remain predictable and avoid introducing challenging-to-debug side effects. Let’s consider a simple example of managing a list of items using the spread operator for immutability:
import React, { useState } from 'react';
const ItemList = () => {
// Local state for the list of items
const [items, setItems] = useState(['Item 1', 'Item 2', 'Item 3']);
// Function to add a new item to the list immutably
const addItem = () => {
// Using the spread operator to create a new array with the additional item
const newItem = `Item ${items.length + 1}`;
setItems([...items, newItem]);
};
return (
<div>
<h2>Item List</h2>
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
<button onClick={addItem}>Add Item</button>
</div>
);
};
export default ItemList;
Using libraries for advanced state management such as Recoil, Redux, or others
As your React JS application evolves and becomes more intricate, the necessity for a robust state management solution may arise. Redux and Recoil stand out as prominent options for overseeing global state, offering potent tools tailored for handling large-scale applications.
However, it’s crucial to introduce these libraries judiciously, reserving them for situations where their advanced capabilities are genuinely required. For smaller projects, the management of state at the local component level might prove to be adequate. Let’s contemplate a scenario where, integrating Redux becomes beneficial due to the complexity of the application. Below is a simplified example demonstrating the setup:
import { createStore } from 'redux';
// Reducer function handling state transitions
const rootReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
// Create the Redux store
const store = createStore(rootReducer);
export default store;
import React from 'react';
import { connect } from 'react-redux';
const Counter = ({ count, increment, decrement }) => {
return (
<div>
<h2>Count: {count}</h2>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
// Connect the Counter component to Redux state and actions
const mapStateToProps = (state) => ({
count: state.count,
});
const mapDispatchToProps = (dispatch) => ({
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' }),
});
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
Memorization techniques for state updates
Reacts reconciliation algorithm, responsible for determining when and how to update the user interface, can become resource-intensive, particularly in applications with a substantial amount of state and intricate UI components.
To mitigate this, leveraging memorization techniques such as React.memo or useMemo becomes crucial. These functions empower you to cache a component’s result based on its dependencies. Thus, easing the burden on Reacts reconciliation process and preventing unnecessary re-rendering.
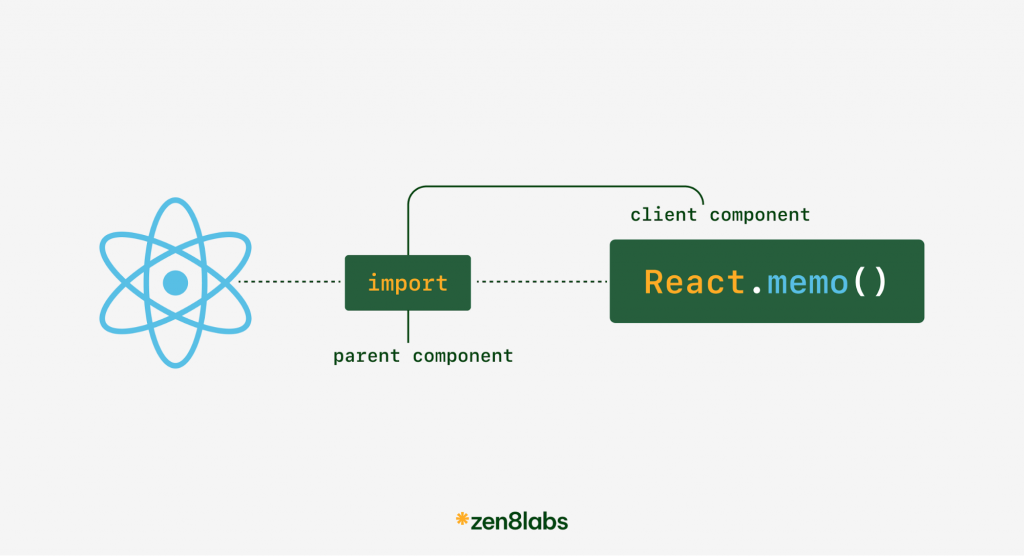
Consider using React Context API
Reacts Context API is a built-in solution for sharing state across components without resorting to a global state management library. It allows you to pass data through the component tree without passing props explicitly at every level.
Context is ideal for smaller applications or when you need to share state between a few closely related components. However, be cautious with using Context, as it can lead to performance issues if overused.
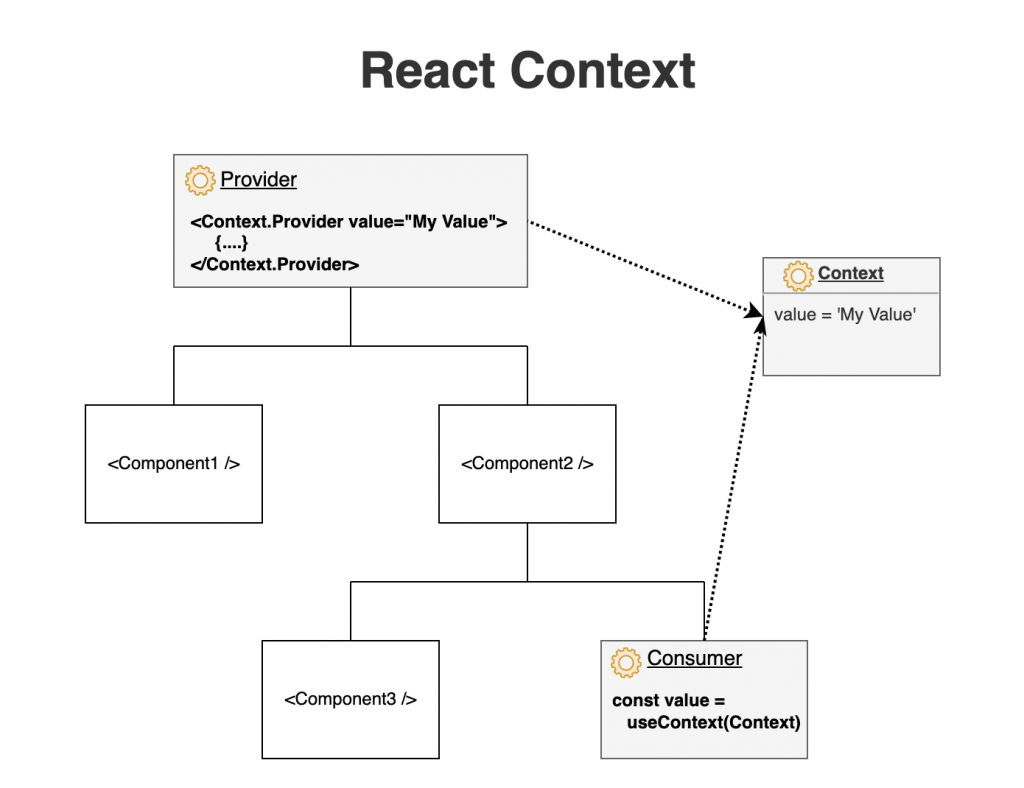
Keep pace with Reacts evolution: Stay updated with the latest features and libraries
React JS evolves with new features and libraries regularly. Stay informed to uphold your app’s performance. These best practices equip you to navigate state management challenges, always emphasizing simplicity and maintainability.
Conclusion
An environment where simplicity, maintainability, and peak performance come together is orchestrated by the symphony of these best practices and strategic insights. With this information at hand, you can take advantage of the full potential of your applications and successfully navigate the constantly changing nuances of React state management.
For more information during your next coding adventure, try us at zen8labs till then, happy coding!
Tuyen Tran, Software Engineer