Concurrency plays a vital role in building modern, responsive, and performant applications. With the introduction of Async/Await in Swift 5.5, developers now have a powerful tool at their disposal to simplify asynchronous programming and handle concurrent tasks more efficiently. In this blog post, brought to you by zen8labs, we will dive deep into mastering concurrency in Swift with Async/Await, exploring its advanced features, best practices, and practical use cases.
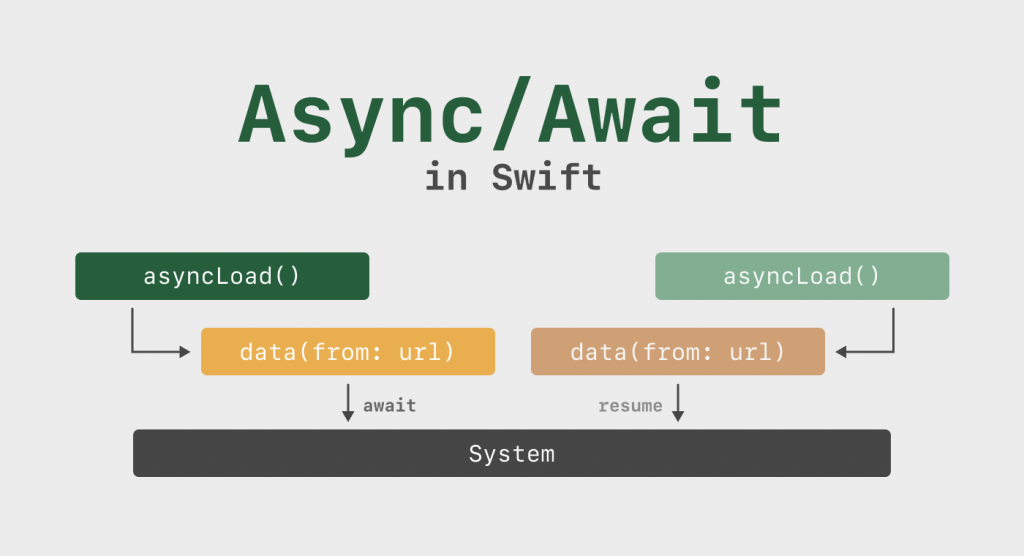
Understanding Async/Await
Before we delve into mastering concurrency with Async/Await, let’s briefly recap its core concepts. Async/Await is a language-level feature that enables developers to write asynchronous code in a more sequential and synchronous style. By marking functions as asynchronous using the async keyword and using the await keyword to pause execution until a result is available, Async/Await eliminates the complexities of nested callbacks and closures, making code more readable and maintainable.
Advanced features of Async/Await
To harness the full power of concurrency in Swift, let’s explore some advanced features of Async/Await.
Structured concurrency
Swift’s Task API provides structured concurrency, allowing you to create and manage concurrent tasks. By using Task.detached or TaskGroup, you can spawn independent tasks that execute concurrently, ensuring proper resource management and error propagation.
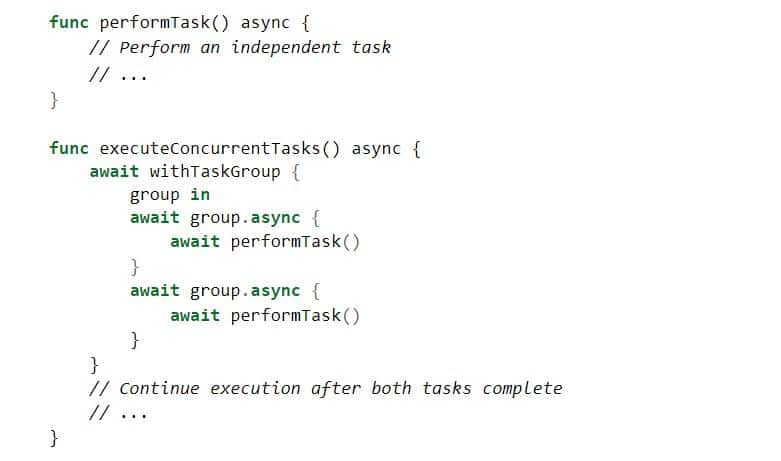
Cancellation
Async/Await supports task cancellation, enabling you to cancel tasks that are no longer needed. By calling task.cancel(), you can gracefully stop task execution and free up system resources.
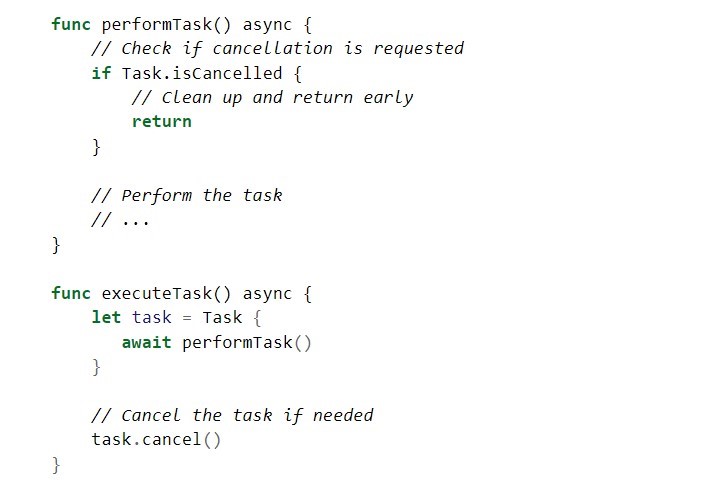
Task prioritization
You can prioritize tasks to ensure critical tasks are executed promptly. By assigning priority levels using Task(priority:), you can control the order in which tasks are executed and optimize resource allocation.
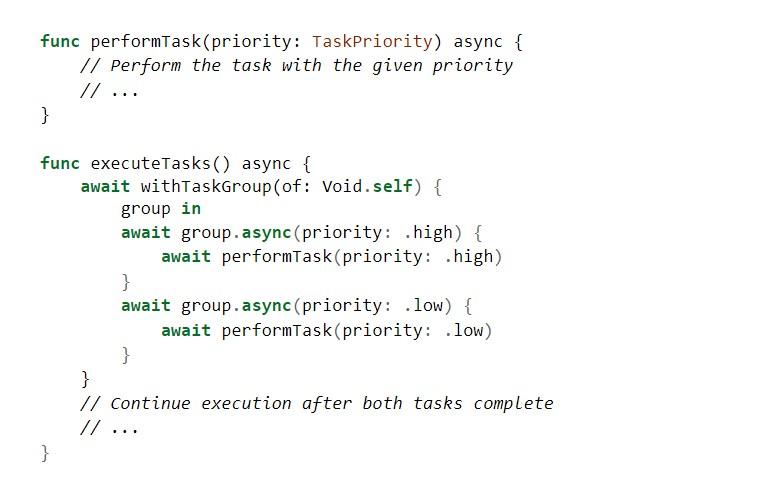
Async sequences
Swift’s async sequences allow you to work with a stream of values asynchronously. You can use AsyncSequence and AsyncIteratorProtocol to iterate over asynchronous sequences, opening new possibilities for working with data streams.
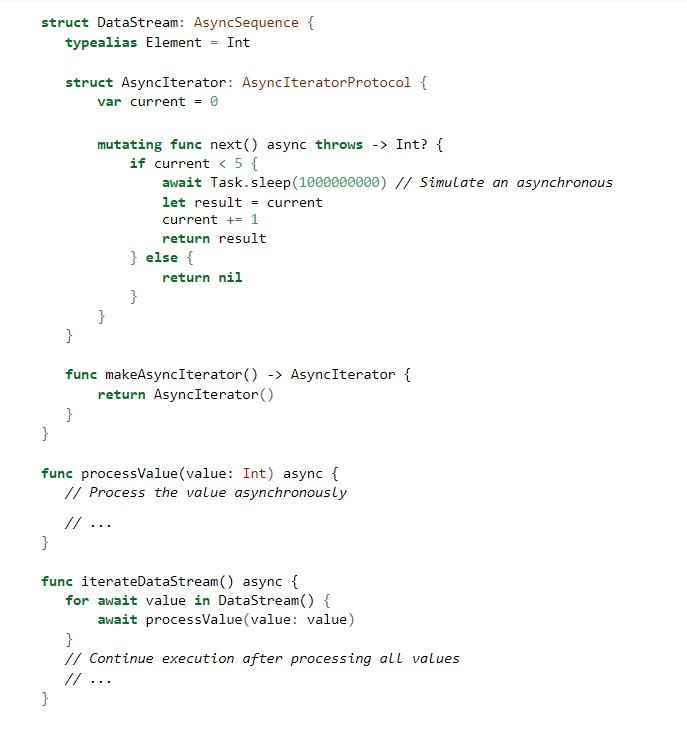
Best practices for mastering concurrency
To master concurrency in Swift with Async/Await, it’s essential to follow some best practices:
- Decompose complex tasks: Break down complex tasks into smaller, more manageable subtasks. This improves code readability, testability, and reusability. Consider using functions, closures, or async sequences to modularize your code.
- Prioritize responsiveness: Concurrency should focus on enhancing the responsiveness of your applications. Identify critical sections that require concurrency and prioritize their execution. This ensures a smooth user experience, especially in UI-related tasks.
- Error handling: Pay careful attention to error handling in Async/Await code. Swift’s structured error handling provides a robust mechanism to catch and propagate errors. Use do-catch blocks to handle errors appropriately and ensure graceful recovery.
- Performance optimization: While Async/Await simplifies concurrency, it’s still important to optimize performance. Profile your code, identify potential bottlenecks, and consider techniques like lazy initialization, caching, and efficient resource management to improve performance.
Practical use cases
Concurrency with Async/Await unlocks a wide range of use cases. Here are a few practical examples:
- Networking: Asynchronous network requests, parallel image downloads, and data processing pipelines can all benefit from the streamlined code structure provided by Async/Await.
- User interfaces: Concurrency enhances UI responsiveness by offloading heavy tasks, such as data loading or image processing, to background threads while keeping the UI thread responsive.
- Data processing: Async/Await simplifies working with large datasets, enabling concurrent data processing, such as filtering, mapping, or reducing operations.
- Multiplatform development: With the advent of SwiftUI and the convergence of iOS and macOS development, Async/Await provides a unified approach to handle asynchronous tasks across multiple Apple platforms.
References
To provide a comprehensive understanding of concurrency in Swift with Async/Await, we have referenced various resources and documentation. Here are some key references that you can explore for further learning:
Swift documentation – Concurrency
Apple’s official documentation on concurrency in Swift provides detailed information about Async/Await, structured concurrency, task cancellation, and more. You can find comprehensive guides, code examples, and API references at https://developer.apple.com/documentation/swift/concurrency.
WWDC videos
Apple’s Worldwide Developers Conference (WWDC) sessions offer valuable insights into Swift concurrency. The following WWDC videos cover Async/Await and related topics:
- “Meet Async/Await in Swift”: This video introduces Async/Await and demonstrates its usage in various scenarios. https://developer.apple.com/videos/play/wwdc2021/10132
- “Swift concurrency: Update a sample app”: Discover Swift concurrency in action https://developer.apple.com/videos/play/wwdc2021/10194/
Swift forums
The Swift Forums are an excellent resource for discussions and community support on Swift concurrency. You can browse topics related to Async/Await, ask questions, and gain insights from experienced developers. Visit https://forums.swift.org/c/development/async-await to explore the Async/Await discussions.
Swift Evolution Proposals
To understand the design decisions and evolution of Async/Await in Swift, you can refer to the Swift Evolution proposals. The proposal SE-0296 “Async/Await” introduces the Async/Await syntax and explains the rationale behind its implementation. Read the proposal at https://github.com/apple/swift-evolution/blob/main/proposals/0296-async- await.md.
Open-source projects and tutorials
Exploring open-source projects and tutorials can provide practical examples and insights into real-world usage of Async/Await in Swift. Websites like GitHub, Swift by Sundell https://www.swiftbysundell.com, and Hacking with Swift
https://www.hackingwithswift.com offer a wealth of resources to deepen your understanding and gain hands-on experience.
Conclusion
Mastering concurrency in Swift with Async/Await empowers developers to build highly responsive, scalable, and efficient applications. By understanding the advanced features, following best practices, and leveraging practical use cases, you can unlock the full potential of Async/Await.
Concurrency is a fundamental aspect of modern application development, and Async/Await in Swift provides a powerful and intuitive way to tackle it. Embrace the benefits of Async/Await, experiment with its advanced features, and continuously refine your approach to concurrency to create exceptional Swift applications.
Happy mastering!
About zen8labs
zen8labs adopts cutting-edge technology practices and tools to ensure alignment of winning business solutions and impactful optimization. A wealth of experience driving efficiencies and innovation allows us to bring that perspective into executing strategic outcomes and building high-performing delivery capability.
Learn more about zen8labs here!
Quan Nguyen, Mobile Engineer