In ReactJS, component communication is a fundamental concept. While parent-child communication is relatively straightforward, what happens when you need to make two sibling components talk to each other without passing props through their parent? At zen8labs, we strive to solve problems in various ways, and this is one of the methods we have applied. That’s the Observer pattern comes into play, and we’ll explore how to implement it using the mitt library.
What is the Observer Pattern?
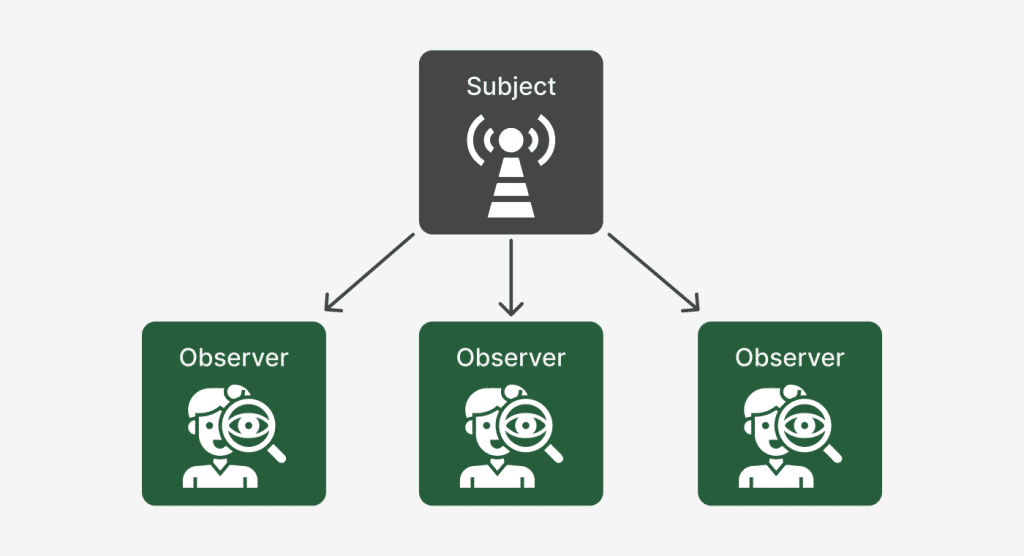
The Observer pattern is a behavioral design pattern that defines a one-to-many dependency between objects. When one object (the subject) changes state, all its dependents (observers) are notified and updated automatically. In the context of React, the subject will be the component that holds the state, and the observers will be the components that need to react to state changes.
Enter `mitt`
`mitt` is a tiny (less than 200 bytes) event emitter library for Node.js and browsers. It’s simple yet powerful and can be a handy tool for implementing the Observer pattern in ReactJS applications.
Installing mitt
First, let’s install mitt into your React project:
npm install mitt
or
yarn add mitt
Setting Up the Observer
Assuming you have two sibling components: ComponentA and ComponentB, and you want them to communicate without going through their parent. Here’s how you can set up the Observer pattern using `mitt`.
In ComponentA:
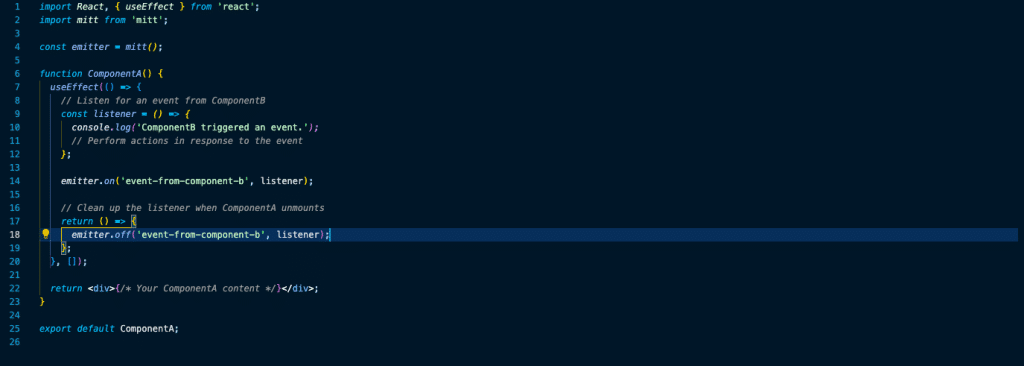
In ComponentB:

In this setup, ComponentB emits an event when a button is clicked. ComponentA listens for this event and responds accordingly. The mitt library facilitates this communication without the need for a parent component to pass props.
Conclusion
The Observer pattern, with the help of the mitt library, provides an elegant solution for inter-component communication in ReactJS applications. It allows sibling components to communicate efficiently without the need for complex prop drilling or context management.
Remember that this isn’t a pattern you should be reaching for as the first solution, as usually there are better ways to handle communication between sibling components. Nevertheless, if you need to communicate directly between adjacent components or maybe even components at different levels in the component tree, you can do so by using the mitt package or rolling out your own observer logic.
Check out other useful Tech insights here!
Nam Do, Head of Learning & Development